Hi James,
The source code of an ARKit demo App from us may be helpful.
Once you understand and can access the depthMapp, you may like to pick a point around the screen center.
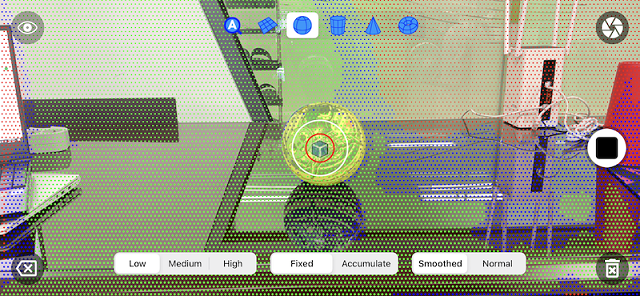
The red circle at the screen center defines the search area for the point you look for. The radius of the red circle is to be given in pixel (of camera image) and to be converted as the vertex angle of a search cone.
func pickPoint(rayDirection ray_dir: simd_float3, rayPosition ray_pos: simd_float3, vertices list: UnsafePointer<simd_float4>, count: Int, _ unitRadius: Float) -> Int {
let UR_SQ_PLUS_ONE = unitRadius * unitRadius + 1.0
var minLen: Float = Float.greatestFiniteMagnitude
var maxCos: Float = -Float.greatestFiniteMagnitude
var pickIdx : Int = -1
var pickIdxExt: Int = -1
for idx in 0..<count {
let sub = simd_make_float3(list[idx]) - ray_pos
let len1 = simd_dot( ray_dir, sub )
if len1 < Float.ulpOfOne { continue; } // Float.ulpOfOne == FLT_EPSILON
// 1. Inside ProbeRadius (Picking Cylinder Radius)
if simd_length_squared(sub) < UR_SQ_PLUS_ONE * (len1 * len1) {
if len1 < minLen { // find most close point to camera (in z-direction distance)
minLen = len1
pickIdx = idx
}
}
// 2. Outside ProbeRadius
else {
let cosine = len1 / simd_length(sub)
if cosine > maxCos { // find most close point to probe radius
maxCos = cosine
pickIdxExt = idx
}
}
}
return pickIdx < 0 ? pickIdxExt : pickIdx
}
There are 3 cases:
- If there are at least 1 depthMap points inside the view cone, the top most point to the camera COP will be chosen.
- Else if there are at least 1 depthMap points outside the view cone, the closest point to the red circle in screen will be chosen.
- Otherwise, there is no point from depthMap (empty depthMap).
By adjusting the radius of the red circle, you can control the precision of picking a point.
CurvSurf