Unfortunately, the two buttons of UIBarButtonItem are also placed incorrectly in the empty test project under iOS 16 if I do everything as in the real project.
I add the view of a second UIViewController to the main view and then create the UINavigationBar on top of the second UIViewController's view. It worked fine until iOS 15.5. Unfortunately not with iOS 16. Does anyone know how I can fix the problem?
UIEdgeInsets safeAreaInsets = self.view.superview.safeAreaInsets;
secondViewController.view.frame = CGRectMake(safeAreaInsets.left,
safeAreaInsets.top,
self.view.frame.size.width - safeAreaInsets.left - safeAreaInsets.right,
self.view.frame.size.height - safeAreaInsets.top - safeAreaInsets.bottom);
secondViewController.view.backgroundColor = [UIColor darkGrayColor];
[self.view addSubview:secondViewController.view];
UINavigationBar *navigationBar = [[UINavigationBar alloc] initWithFrame:CGRectMake(0, 0, secondViewController.view.frame.size.width, 44)];
[secondViewController.view addSubview:navigationBar];
UINavigationItem *navigationItem = [[UINavigationItem alloc] init];
navigationItem.leftBarButtonItem = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemCancel target:self action:nil];
navigationItem.rightBarButtonItem = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemDone target:self action:nil];
[navigationBar pushNavigationItem:navigationItem animated:NO];
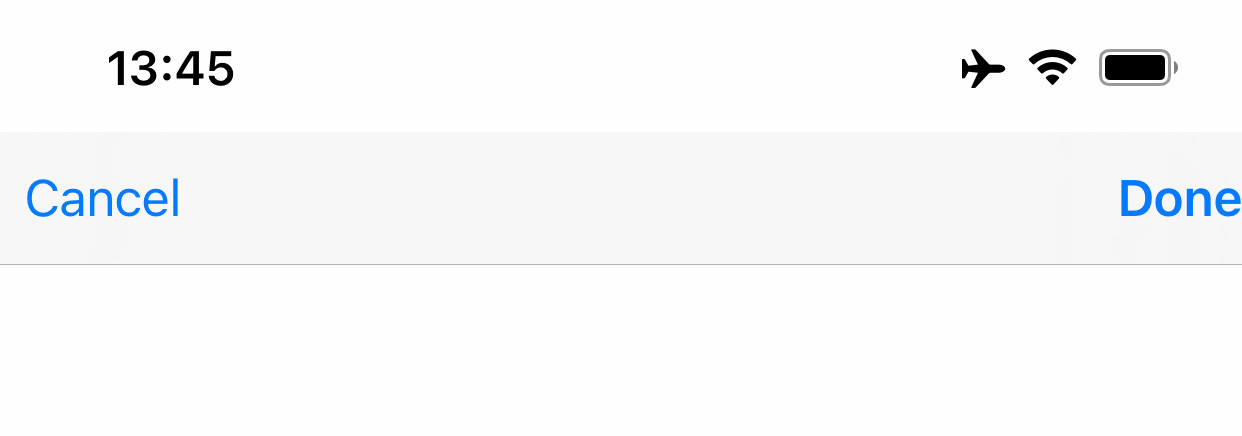
With the code below it looks good even with iOS 16, but I really need the first method as in the code above.
UIEdgeInsets safeAreaInsets = self.view.superview.safeAreaInsets;
secondViewController.view.backgroundColor = [UIColor whiteColor];
UINavigationBar *navigationBar = [[UINavigationBar alloc] initWithFrame:CGRectMake(0, safeAreaInsets.top,
secondViewController.view.frame.size.width, 44)];
[secondViewController.view addSubview:navigationBar];
UINavigationItem *navigationItem = [[UINavigationItem alloc] init];
navigationItem.leftBarButtonItem = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemCancel target:self action:nil];
navigationItem.rightBarButtonItem = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemDone target:self action:nil];
[navigationBar pushNavigationItem:navigationItem animated:NO];
secondViewController.modalPresentationStyle = UIModalPresentationFullScreen;
[self presentViewController:secondViewController animated:NO completion:nil];
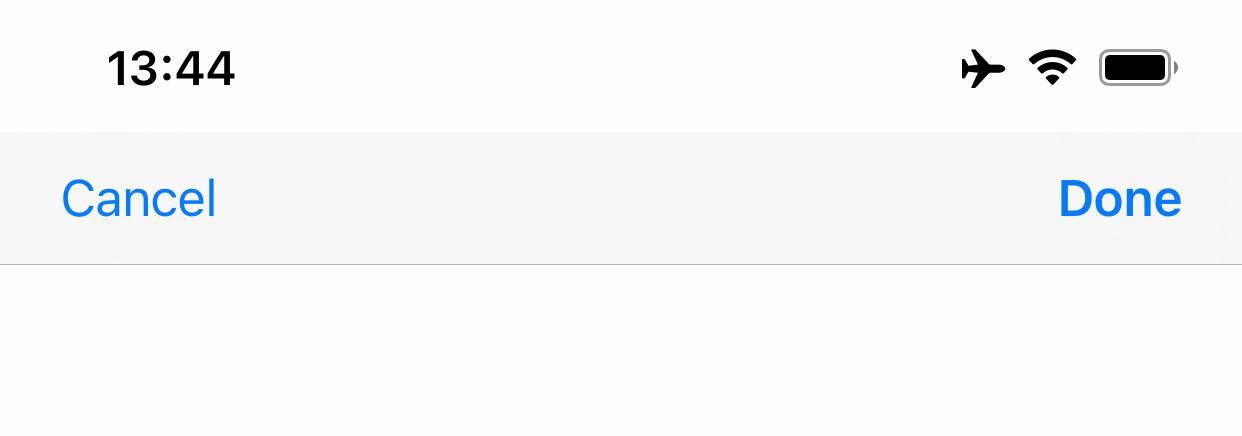