Hi, Claude31, thank you for the answer.
So, the model looks like this:
class Item {
var name: String = ""
@Relationship(deleteRule: .cascade, inverse: \Picture.item)
var pictures: [Picture] = []
init(name: String = "", pictures: [Picture] = []) {
self.name = name
self.pictures = pictures
}
}
@Model
class Picture {
@Attribute(.externalStorage)
var imageData: Data?
var thumbNailData: Data?
var item: Item?
init(imageData: Data? = nil, thumbNailData: Data? = nil, item: Item? = nil) {
self.imageData = imageData
self.thumbNailData = thumbNailData
self.item = item
}
}
And the View looks like this:
struct ContentView: View {
@Query private var items: [Item]
var body: some View {
NavigationStack {
List {
ForEach(items) { item in
HStack {
if !item.pictures.isEmpty, let pic = item.pictures.first?.thumbNailData, let uiImage = UIImage(data: pic) {
Image(uiImage: uiImage)
.resizable()
.aspectRatio(contentMode: .fill)
.frame(width: 80, height: 80)
.clipShape(.rect(cornerRadius: 10))
}
Text(item.name)
}
}
}
.navigationTitle("Items: \(items.count)")
}
}
}
As it appears, it already gets over 300 MB of memory
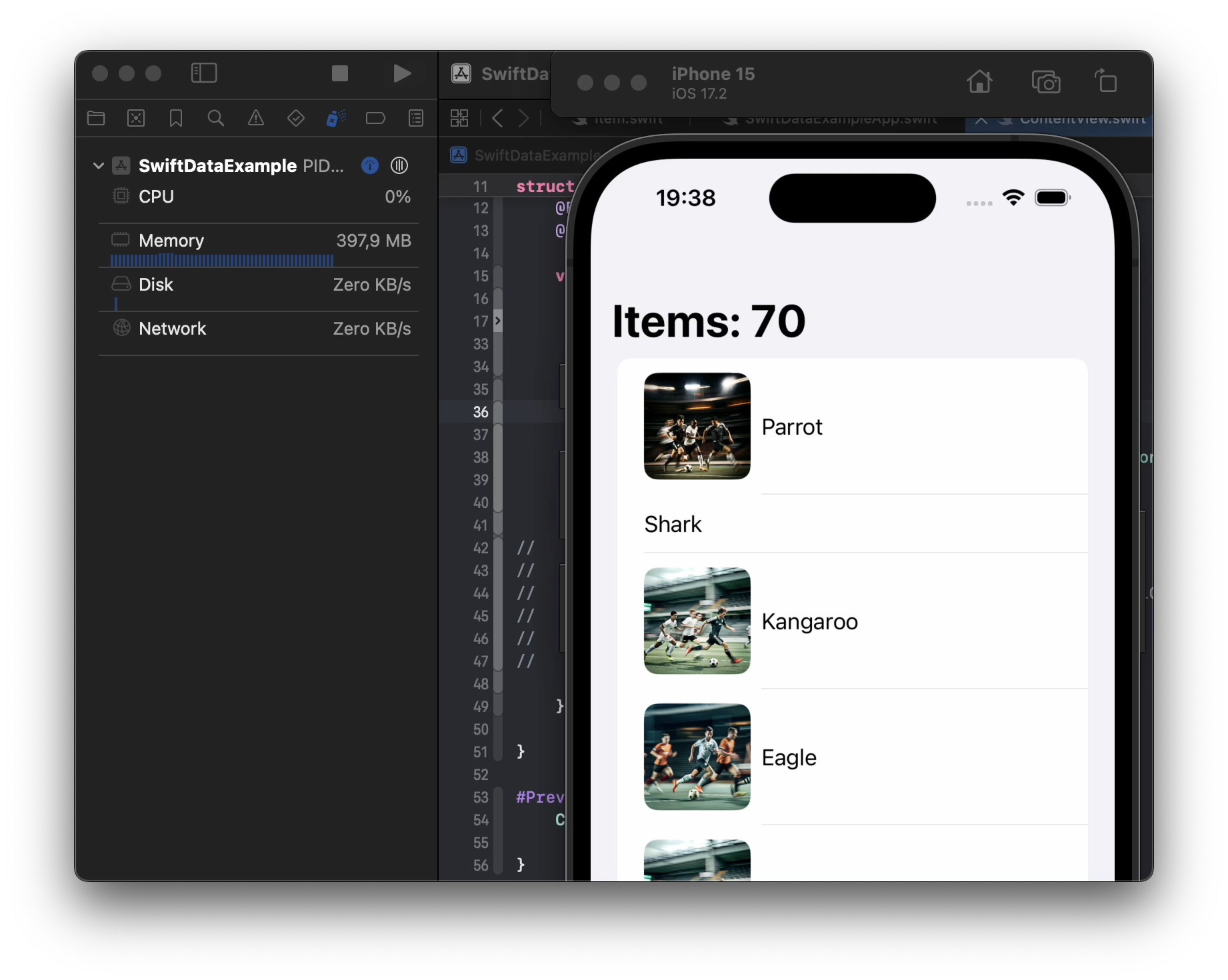
and while it scrolls, it takes over 3 GB and never goes down.
So am I doing something in a wrong way?