In a project using Core Data where I pass multiple objects to the navigationDestination (see example below), I'm seeing a lot of "Update NavigationAuthority possible destinations tried to update multiple times per frame."
when I navigate in the Stack. After few push and pop in the stack, the app freezes, the memory increases, the CPU runs at 100%, then the app crashes. I checked the logs from my device: the issues are different every time, but it seems to be linked to a UI update.
.navigationDestination(for: Paragraph.self) { paragraph in
ParagraphDetail(
post: post,
paragraph: paragraph) // -> Update NavigationAuthority possible destinations tried to update multiple times per frame.
}
In a NavigationStack where I only pass one object in the navigationDestination (see below), I don't have this log message, and the app never freezes.
.navigationDestination(for: Paragraph.self) { paragraph in
ParagraphDetailOnlyParagraph(paragraph: paragraph)
}
To identify if it's related to Core Data or not, I reproduced the same NavigationStack with plain Structs, or simple Class objects. I don't see the log message, even when I pass multiple objects to the navigationDestination. The app never freezes.
I'll file a FB. It seems to be a bug in beta 1.
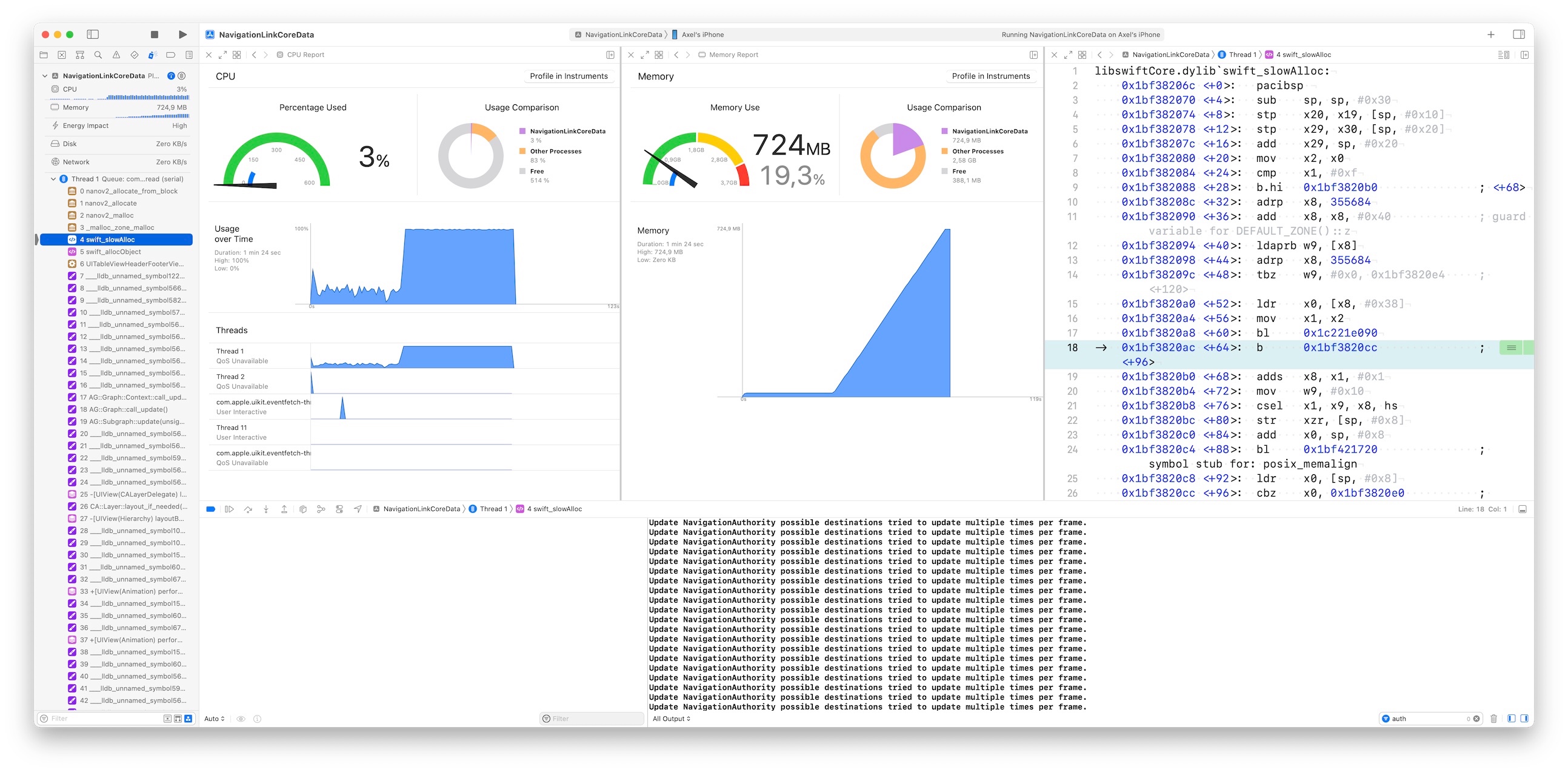
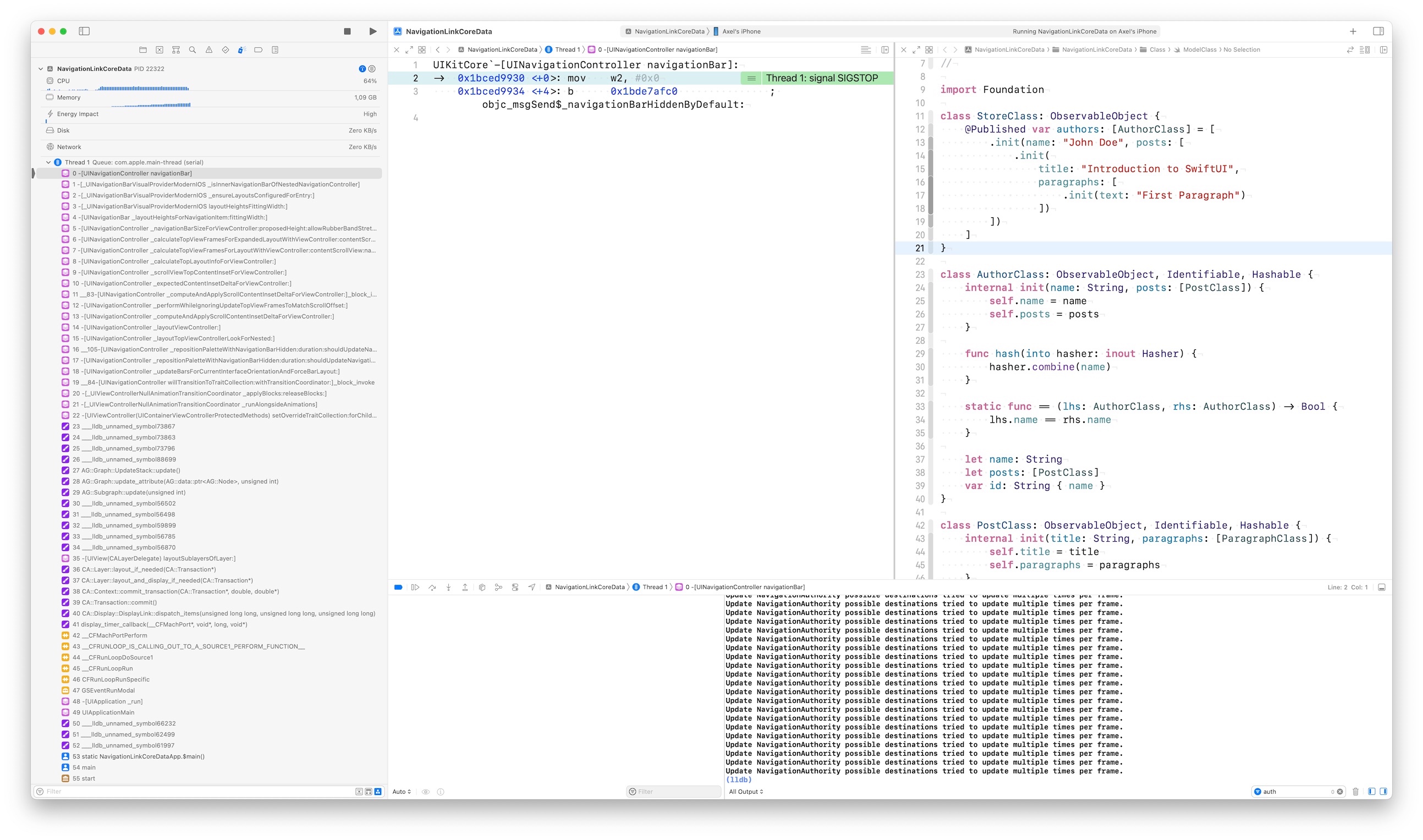