This is the whole function used to load and start the music kit instance:
const loadAppleMusic = async () => {
const mk = await (window as any).MusicKit;
console.log("mk", mk);
const token = await getAppleDevToken();
await mk.configure({
developerToken: token,
app: {
name: "MusicKit Music Example",
build: "1.0.0",
},
});
musicKit.value = mk.getInstance();
musicKit.value.volume = 0.5;
};
I also previously had it as:
musicKit.value = await mkConfigure({})
Then I use this function to play the songs by their id:
const loadDynamicTracks = async () => {
await musicKit.value.setQueue({
songs: ["1616228595", "1564530724"],
startPlaying: true,
});
};
I also added these event listeners:
musicKit.value.addEventListener("queueIsReady", (data: any) => {
console.log("Queue is ready", data);
});
musicKit.value.addEventListener("queueItemsDidChange", (data: any) => {
console.log("Queue items changed", data);
});
These fire off once everything is loaded, and it looks like I'm getting the proper songs in the queue from what I've seen. Is there something else I need to update? Seems like I'm missing something that would move to the next song in the queue. Is there an event listener I should add that would help me troubleshoot this? I added this one as well, but it doesn't get called:
musicKit.value.addEventListener("mediaPlaybackError", (data: any) => {
console.log("Playback Error", data);
});
I appreciate the help.
Also this is what I'm getting in the console:
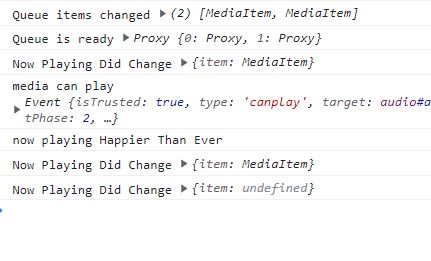
Right after the song plays "now playing" shows undefined for the item. As you can see in the Queue items changed there are two media items so I don't know what's going on.